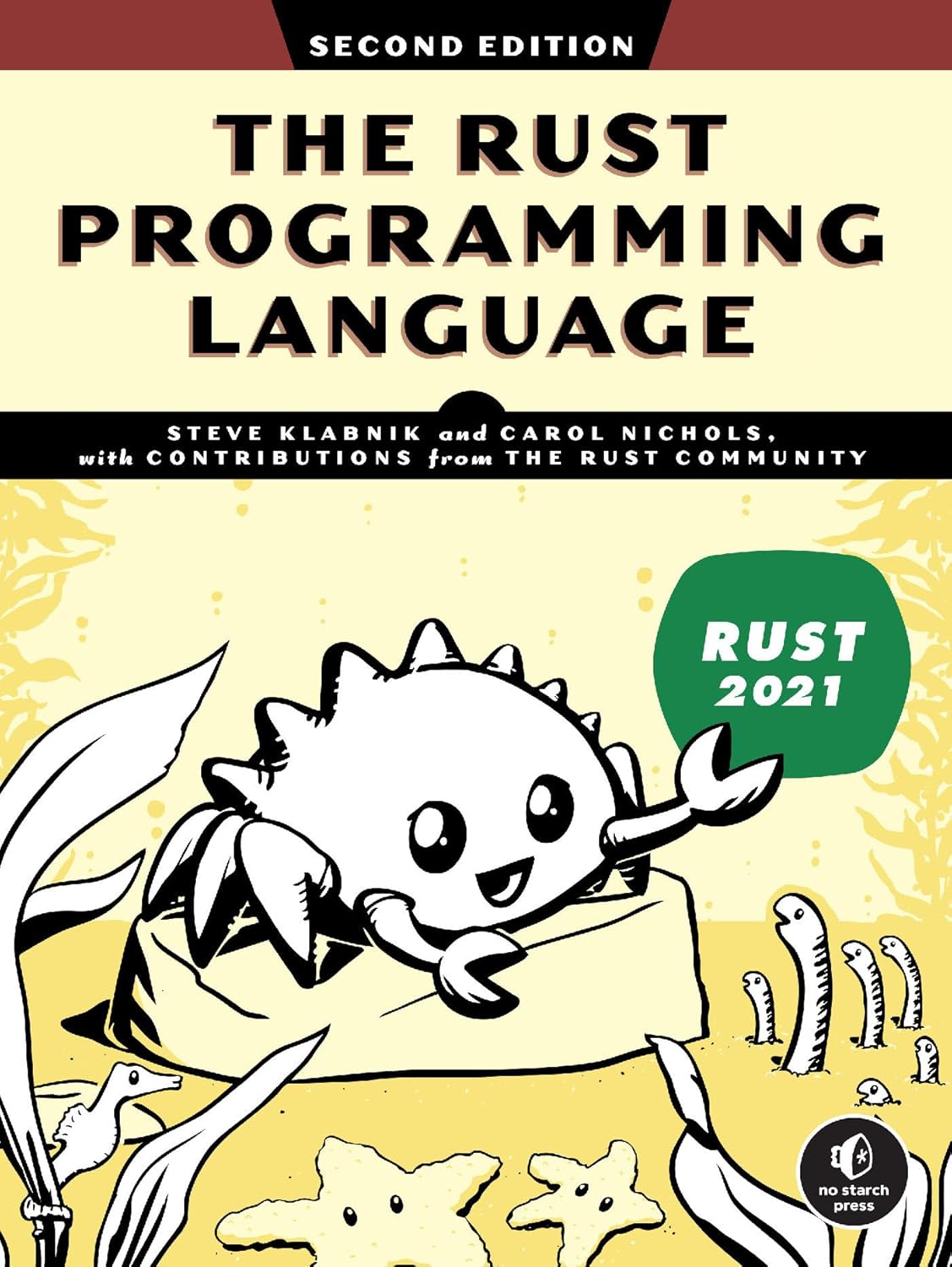
What does it mean to be an effective programmer in today’s ever-evolving tech landscape? One language that continues to gain traction among developers is Rust, and I recently had the opportunity to read “The Rust Programming Language, 2nd Edition.”
Understanding Rust
Rust is known for its speed, memory safety, and concurrency. If you’re like me, these features are incredibly appealing, especially when building applications that demand performance and reliability. I found that this book does an excellent job of introducing these concepts in a way that feels approachable, regardless of my programming background.
Why Rust is Gaining Popularity
One of the first things I noticed about Rust is its ability to prevent common programming errors. For someone who has experienced the frustration of debugging memory leaks or segmentation faults, Rust’s compile-time checks are a breath of fresh air. The language encourages a different way of thinking about data safety, which, I must say, can be a game-changer in my coding journey.
The Structure of the Book
The book is structured in a user-friendly manner, beginning with the basics and gradually building up to more advanced topics. This progressive approach is something I appreciated. It allowed me to solidify my understanding before tackling more complex subjects.
Chapters Breakdown
Here’s a general breakdown of the chapters, which adds clarity to how the content is organized:
Chapter | Topic | Description |
---|---|---|
1 | Getting Started | An introduction to Rust, setup, and basic syntax. |
2 | Programming a Guessing Game | Creating a simple game to understand functions. |
3 | Understanding Ownership | The core feature of Rust that ensures memory safety. |
4 | Structs and Enums | Learning to create custom data types. |
5 | Modules and Packages | Organizing code into reusable components. |
6 | Error Handling | Techniques for managing errors gracefully. |
7 | Generics | Writing flexible functions and types. |
8 | Traits | Utilizing interfaces to define shared behavior. |
9 | Lifetimes | Understanding scope and memory management. |
This table is a quick reference guide for anyone looking to navigate the book. I found that constantly referring back to this framework helped me keep track of my progress.
Getting Started with Rust
When I started with the first chapter, it felt like a gentle introduction. The author, Steve Klabnik, and Carol Nichols, really made the setup process straightforward. Setting up Rust on my machine didn’t take long, and I appreciated that they included installation instructions for various operating systems.
Syntax and Basics
Learning the syntax was both refreshing and familiar. For me, coming from a background in Python, the syntax felt a bit stricter, which I appreciated. The emphasis on type annotations and clear variable declarations nudged me towards writing cleaner, more maintainable code.
Building a Simple Project
Progressing through the book, I enjoyed the chapter that guided me to build a simple guessing game. This hands-on project was such a great way to consolidate what I had learned so far. Crafting the game allowed me to understand key concepts such as functions, loops, and control flow in a practical situation.
Functions in Rust
I found the way Rust handles functions to be both intuitive and strict. Any programmer can relate to the significance of writing reusable code, and Rust encourages that through its structured function definitions. I liked the clear demarcation of an entry point in a program, along with the requisite scoping rules.
Mastering Ownership
I can’t stress enough how vital the concept of ownership is within Rust. It sets Rust apart from other languages I’ve worked with. This chapter dives deep into ownership, borrowing, and lifetimes, which are crucial for managing memory safely.
Ownership and Borrowing
Getting my head around ownership was challenging at first, but it began to click as I worked through practical examples. The idea that each value in Rust has a single owner creates a clear pathway for understanding how data is stored and accessed in memory. Borrowing, on the other hand, opens up a plethora of possibilities for allowing multiple references to a value, which I found invaluable.
Lifetimes Defined
The concept of lifetimes was initially daunting, but the way it was broken down into relatable examples made it much easier to grasp. Understanding how lifetimes relate to function parameters and return types allowed me to write code that is not only efficient but also safe from data races.
Structs and Enums
Moving on to structs and enums, I found myself really appreciating how Rust encourages the use of these data structures. Creating structured data with structs felt natural, and learning about enums for defining type variants was pretty enlightening.
Custom Data Types
Being able to define custom types is essential for any language, and Rust makes that easy. The author’s thorough explanations of how to implement these types, along with practical examples, ensured I was comfortable with using them.
Modules and Packages
The chapters covering modules and packages helped me understand how to structure my projects effectively. Organizing code into manageable units is something I value tremendously in programming.
Creating Reusable Code
The discussion on modules showed me the importance of encapsulation. It’s important to keep related functions and structures together, and the book provides clear guidelines on how to achieve that. With Rust’s module system, I felt better equipped to write reusable and maintainable code.
Error Handling
Rust encourages handling errors in a way that contrasts with many other programming languages. I appreciated that the authors did not shy away from discussing this vital aspect.
Result and Option Types
The Result and Option types are brilliant abstractions for error handling. I found that using these types encouraged me to think about what could go wrong in my programs, leading to safer code. By forcing me to address potential errors, Rust pushed me toward writing more robust applications.
Generics
Generics were another fascinating topic that I could see elevating my coding practices. Learning how to write flexible functions that work with various data types was refreshing.
Writing Flexible Code
This chapter taught me that generics are essential for building libraries and frameworks in Rust. I was able to create more abstract and reusable functions that can accommodate different types without sacrificing type safety.
Traits
Traits are essentially Rust’s answer to interfaces, and I found this concept very powerful. Learning how they can be employed to define shared functionality across types was eye-opening.
Implementing Behavior
By implementing traits, I could ensure that various types in my program could be treated similarly, promoting code reuse and modular design. This understanding served as a critical foundation as I progressed into more complex scenarios.
Lifetimes Recap
This part of the book reiterated the importance of lifetimes in cases involving complex data structures. Realizing how lifetimes can appear in function signatures solidified my grasp on Rust’s powerful memory model.
Practical Lifetimes
Lifetimes aren’t just theoretical; they have practical implications. Understanding how they apply to various scenarios helped me avoid common pitfalls, especially when dealing with references in structs and functions.
Advanced Topics
As I breezed through the last few chapters, I encountered several advanced topics that really encourage deep thinking around programming concepts. The discussions about macros and unsafe Rust were quite fascinating.
Workflow and Macros
Rust’s macro system simplifies repetitive tasks and creates a more straightforward coding experience. I appreciated the coverage of both declarative and procedural macros in this section. It was useful to see how macros can enhance my coding efficiency while still adhering to Rust’s safety guarantees.
Unsafe Code
The concept of writing unsafe code felt serious but necessary. In Rust, it serves as a reminder that while you can perform actions that bypass certain safety checks, you have to be aware of the risks involved. This chapter prepared me for those situations when I need fine-tuned control over safety guarantees in exchange for performance.
Conclusion
In the end, “The Rust Programming Language, 2nd Edition,” was more than just a programming book for me. It served as a comprehensive guide to grasping the essence of Rust while providing practical examples that kept me engaged. The authors are seasoned professionals who care deeply about teaching, and their approach goes above and beyond to make this book a must-read.
The insights I gained about memory safety, ownership, and performance truly set Rust apart and have already begun to influence my coding style in other languages as well.
If you’re looking to step into the world of Rust or sharpen your existing skills, I highly recommend picking up this book. It’s more than a resource; it’s an invitation to think differently about how I write code and tackle programming challenges. Rust isn’t just a language; it’s a mindset, and this book lays the groundwork brilliantly.
Disclosure: As an Amazon Associate, I earn from qualifying purchases.